Example: Time in Earth’s Shadow#
A satellite is in a 500 km by 5000 km orbit with its apse line parallel to the line from the earth to the sun, as in Fig. 56. Find the time that the satellite is in the earth’s shadow if:
the apogee is toward the sun
the perigee is toward the sun
Fig. 56 shows the shaded and sunlit regions of the orbit. The satellite will be in shade when its orbit intersects the lines at the edge of the earth, on the other side from the sun. When apogee is towards the sun, the satellite is in the shade from \(a\) to \(b\), and when perigee is towards the sun, the satellite is in the shade from \(c\) to \(d\).
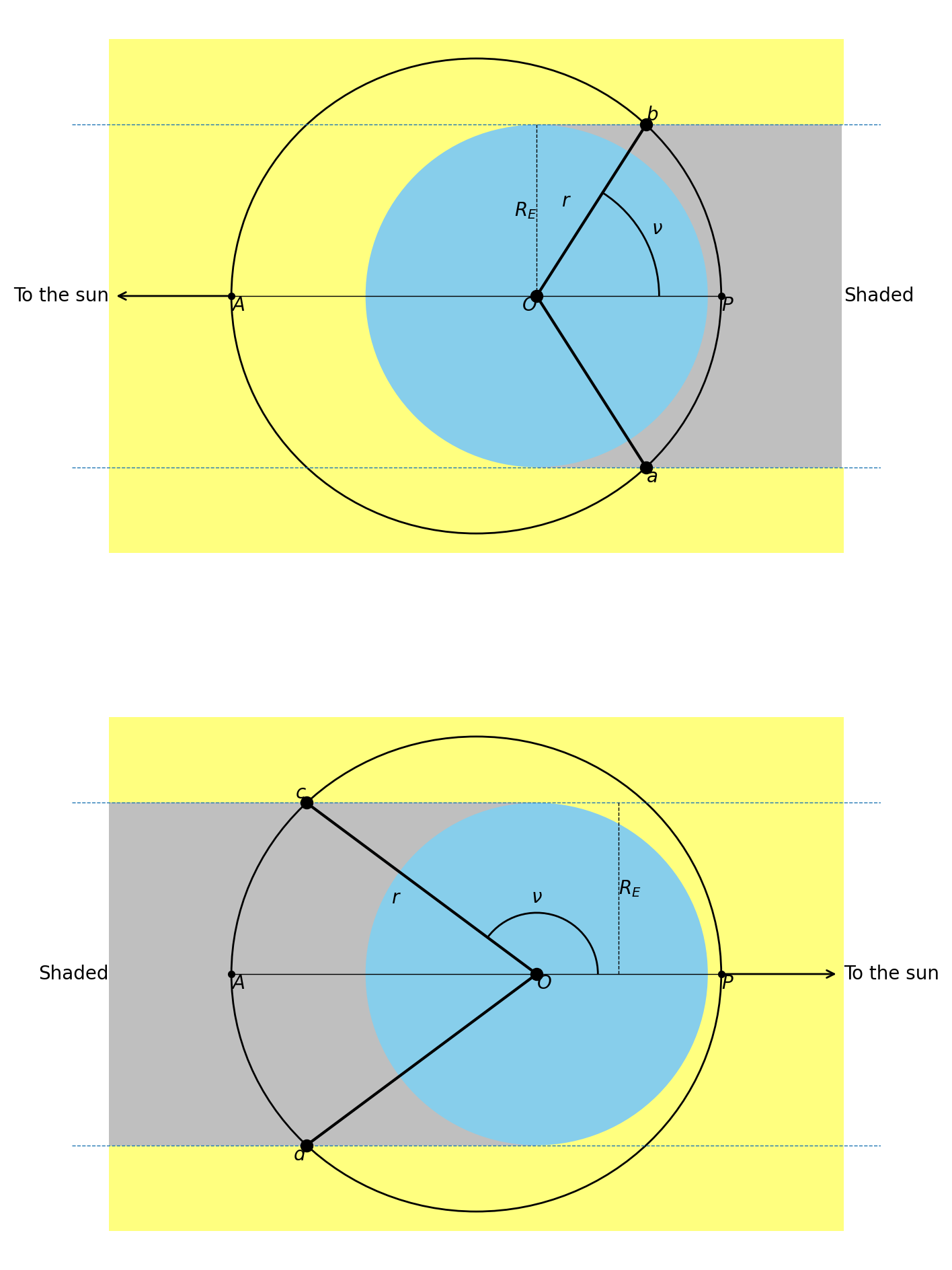
Fig. 56 The orientation of the sun relative to the earth in this example.#
Solution#
In this problem, we are looking for the time the satellite spends between two points in the orbit. We can find the true anomaly of the sun/shadow crossing points geometrically, so this problem is the type where \(\nu\) is given and we are finding \(t\).
We start by finding \(e\), \(a\), and \(T\).
import numpy as np
from scipy.optimize import newton
mu = 3.986004418E5 # km**3/s**2
r_p = 6378 + 500 # km
r_a = 6378 + 5000 # km
R_E = 6378 # km
e = (r_a - r_p) / (r_p + r_a)
a = (r_a + r_p) / 2
T = 2 * np.pi / np.sqrt(mu) * a**(3/2)
The eccentricity of the orbit is \(e =\) 0.2465, the semimajor axis distance is \(a =\) 9128.00 km, and the period is \(T =\) 2.41 hr. Then, we need to solve for the value of \(\nu\) at \(b\) and \(c\). With this value of \(\nu\), we can find the time to fly between the two points. This will tell us the time the satellite is in the shade.
From Fig. 56, we can draw a right triangle from the center of Earth vertically up, the over to the spacecraft, then back down the \(\vector{r}\) vector to the center of the earth. This gives:
However, we don’t know \(r_a\) or \(r_b\) to be able to find \(\nu\). We need another equation, and the orbit equation Eq. (113) will serve.
Now, we can set Eq. (113) and Eq. (215) equal to each other and solve for \(\nu\). We end up with a complicated equation for \(\nu\):
It is possible to solve this equation analytically for \(\nu\), but frankly, I don’t know that much trigonometry. So we will solve this numerically.
We need to find out how many roots to expect in this equation. One way to do that is to plot the equation over a suitable range and see how many times it crosses zero. The suitable range for this problem is \([0, 2\pi]\).
def shadow(nu, e, a, R_E):
"""This function computes the angle 𝜈 in the shadow example problem.
The arguments are the angle, the eccentricity, the semimajor axis, and
the radius of the earth.
"""
return e * np.cos(nu) - (1 - e**2) * a / R_E * np.sin(nu) + 1
nu_range = np.linspace(0, 2 * np.pi)
shadow_result = shadow(nu_range, e, a, R_E)
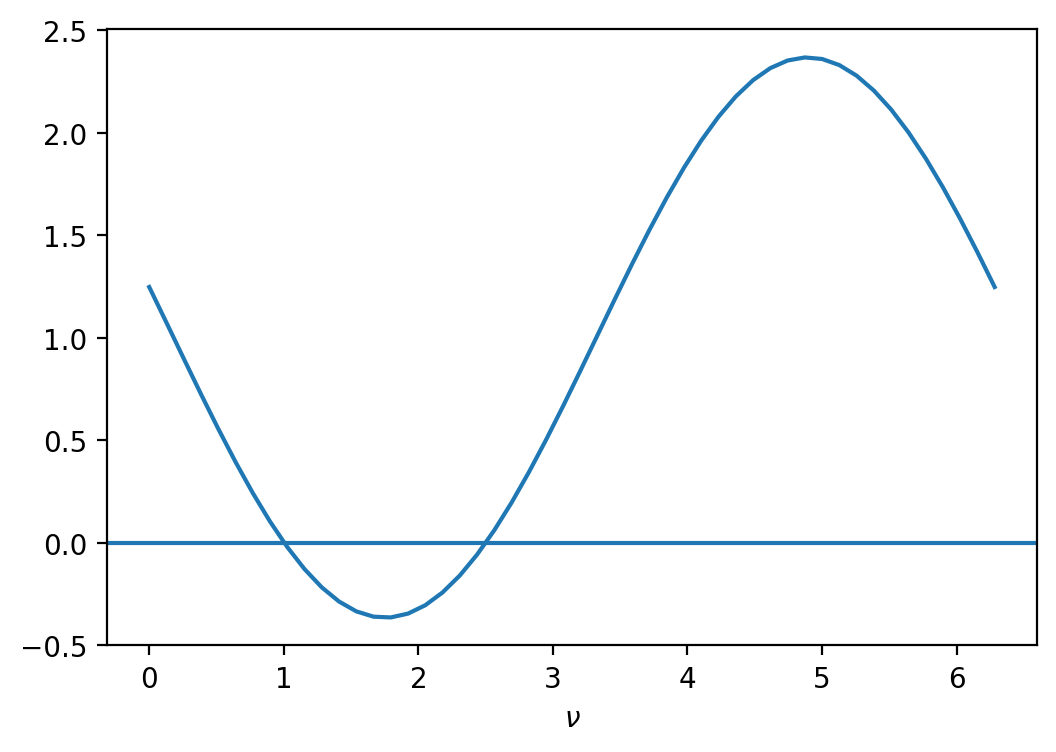
From Fig. 57, we can see there are two roots, one near \(\nu =\) 1 and one near \(\nu =\) 2.5, both in radians. Now we can use scipy.optimize.newton()
to solve the equation. Since we know the approximate roots from Fig. 57, we can use those as initial guesses for the result. Examining Fig. 56, we see that \(\nu \approx\) 1 radians, or approximately 60°, corresponds to point b. \(\nu \approx\) 2.5, or approximately 140°, corresponds to point c.
nu_b = newton(func=shadow, x0=1, args=(e, a, R_E)) # rad
nu_c = newton(func=shadow, x0=2.5, args=(e, a, R_E)) # rad
glue("ellipse-time-in-shadow-nu_b", np.degrees(nu_b))
glue("ellipse-time-in-shadow-nu_c", np.degrees(nu_c))
The true anomaly at point b is \(\nu_b =\) 57.42° and at point c is \(\nu_c =\) 143.36°. Now we can use these values of \(\nu\) to solve for \(E\), then for the mean anomaly, and then for the time since perigee.
E_b = (2 * np.arctan(np.sqrt((1 - e) / (1 + e)) * np.tan(nu_b / 2)))
M_eb = E_b - e * np.sin(E_b)
t_b = M_eb * T / (2 * np.pi) # s
time_in_shadow_ab = 2 * t_b # s
The time to fly from perigee to point \(b\), 14.4 min, is the same as the time to fly from point \(a\) to perigee, because the orbit is symmetrical around the apse line. Therefore, the time the satellite is in shadow when apogee is towards the sun is a little less than half an hour, 28.9 min.
When perigee is towards the sun, then the satellite will be in shadow from point \(c\) to point \(d\). The time the satellite spends in the sun, going from perigee to apogee, is the time flying from perigee to point c. The total time in the sun is twice this time, and the time in shadow is the total period minus the time in the sun.
E_c = (2 * np.arctan(np.sqrt((1 - e) / (1 + e)) * np.tan(nu_c / 2)))
M_ec = E_c - e * np.sin(E_c)
t_c = M_ec * T / (2 * np.pi)
time_in_shadow_cd = T - 2 * t_c
The total time in shadow is 45.3 min when perigee points towards the sun. This result is intuitively correct because the satellite is travelling slower near apogee, due to Kepler’s second law (equal areas in equal times).